# API Documentation
REST API version 1.0 provides programmatic access to the trading engine and interaction with the exchange. Our API Endpoints are fully compatible with CoinMarketCap and CoinGecko digital asset platforms.
# API Base Endpoint
REST https://YOUR_EXCHANGE_URL/api/v1
# DateTime Format
All timestamps are returned to ISO 8601 format or UNIX timestamp in milliseconds (UTC). Example: "2021-05-03T10:20:49.315Z" or "1611715822100".
# Date Format
Some timestamps are returned to ISO 8601 format which includes a calendar date only.
Example: "2022-05-03".
# Number Format
All finance data, e.g., price, quantity, fee, etc., should be arbitrary precision numbers and have a string representation.
Example: "24400.103508".
# API Rate Limits
The following Rate Limits are applied for the REST API interaction:
- for the Market data and other public endpoint requests, the limit is 5 requests per second for one IP;
- for Trading, the limit is 10 requests per second for one user. Trading includes place and cancel order requests;
- for all other requests, the limit is 5 requests per second for one user.
Repeatedly exceeding the Rate Limits can lead to suspension from our API services.
# HTTP Status Codes
All error responses from our REST API have errors
and human readable message
fields. Some errors may contain an additional description
field.
Here are HTTP Status codes:
- 200 OK. Successful request
- 400 Bad Request. Returns JSON with the error message
- 401 Unauthorized. Authorization is required or has been failed
- 403 Forbidden. Action is forbidden
- 404 Not Found. Data requested cannot be found
- 422 Unprocessable Content. Validation failed
- 429 Too Many Requests. Your connection has been rate limited
- 500 Internal Server. Internal Server Error
- 503 Service Unavailable. Service is down for maintenance
- 504 Gateway Timeout. Request timeout expired
# Best Practices
Our development team always tries to bring the best trading experience to our API users. This section contains information about best practices for using the API.
# HTTP Persistent Connection
The TCP connection is kept active for all API requests/responses. Subsequent requests will result in reduced latency.
If you use the HTTP 1.0 client, please ensure it supports the Keep-Alive directive and submit the "Connection: Keep-Alive" header with a request.
Keep-Alive is a part of the HTTP/1.1 or HTTP/2 protocol and enabled by default on compliant clients. However, you will have to ensure your implementation does not set other values as the connection header.
# Public Endpoints
# Server Time
GET /server-time
Retrieves the server time
{
"time": "2022-05-04T11:43:07.107138Z"
}
# Markets
GET /spot/markets
Returns the actual list of available market pairs.
[
{
"trading_pairs": "BTC-USDT",
"base_currency": "BTC",
"quote_currency": "USDT",
"last_price": "28584.63",
"lowest_ask": "28184.63",
"highest_bid": "28981.63",
"base_volume": "373.86241333",
"quote_volume": "11967732.26",
"price_change_percent_24h": "1.70",
"highest_price_24h": "28735.29",
"lowest_price_24h": "28035.35"
},
{
"trading_pairs": "ETH-USDT",
"base_currency": "ETH",
"quote_currency": "USDT",
"last_price": "1747.97",
"lowest_ask": "1713.04",
"highest_bid": "1787.42",
"base_volume": "582.1686",
"quote_volume": "486459.90",
"price_change_percent_24h": "-2.95",
"highest_price_24h": "1749.93",
"lowest_price_24h": "1734.74"
},
...
]
# Currencies
GET /spot/assets
Returns the actual list of available currencies, tokens, etc.
[
{
"name": "Ethereum",
"unified_cryptoasset_id": 346212,
"can_withdraw": true,
"can_deposit": true,
"min_withdraw": "0.01000000",
"max_withdraw": "100000.00000000",
"maker_fee": 0.1,
"taker_fee": 0.25
},
{
"name": "Tether USDT",
"unified_cryptoasset_id": 346214,
"can_withdraw": true,
"can_deposit": true,
"min_withdraw": "10.00000000",
"max_withdraw": "1000000.00000000",
"maker_fee": 0.1,
"taker_fee": 0.25
},
{
"name": "Bitcoin",
"unified_cryptoasset_id": 346225,
"can_withdraw": true,
"can_deposit": true,
"min_withdraw": "0.0010000",
"max_withdraw": "1000.00000000",
"maker_fee": 0.1,
"taker_fee": 0.25
}
...
]
# Ticker
GET /spot/ticker
Returns ticker information.
[
{
"BTC-USDT": {
"last_price": "28584.63",
"base_volume": "370.86241",
"quote_volume": "1419677.26",
"isFrozen": 0
}
},
{
"ETH-USDT": {
"last_price": "1747.97",
"base_volume": "2448.1686",
"quote_volume": "484649.90",
"isFrozen": 0
}
}
]
# Order Books
GET /spot/orderbook
Parameters:
market_pair - String - Name of the market
depth - Numeric - Limit Orders
Get available Order Books
{
"timestamp": 1571911312949,
"bids": [
[
"28583.98000000",
"0.00193000"
],
[
"28584.59000000",
"0.00802000"
],
[
"28583.53000000",
"0.00066000"
],
....
],
"asks": [
[
"28586.94000000",
"0.01748000"
],
[
"28584.99000000",
"0.17756000"
],
[
"28584.98000000",
"0.00664000"
],
....
]
}
# Trades
GET /spot/trades
Parameters:
market_pair - String - Name of the market
limit: (e.g. 15) (optional)
startDate: 2021-01-01 09:12:54 (optional)
endDate: startDate: 2021-01-01 09:12:54 (optional)
Returns trade information for specified market.
[
{
"trade_id": "ea1f73314272eea2454fdd6c20f63db4",
"price": "28584.63000000",
"base_volume": "0.00773000",
"quote_volume": "0.00773000",
"type": "sell",
"timestamp": 1422586698000
},
{
"trade_id": "5cf7f0dd79e5482a7828af2af0eaef05",
"price": "28590.62000000",
"base_volume": "0.00852000",
"quote_volume": "0.00852000",
"type": "sell",
"timestamp": 1422586697000
},
{
"trade_id": "09c1a475d4469ed766a9067ecde41b0b",
"price": "28590.62000000",
"base_volume": "0.00650000",
"quote_volume": "0.00650000",
"type": "sell",
"timestamp": 1422586696000
},
...
]
# Klines
GET /spot/klines
Parameters:
symbol - String - Name of the market
resolution: 15 (interval, e.g. '1', '5', '15', '30', '60', '240', '720', '1D', '3D', '1W', '1M')
from: (start time) (e.g. 1702586314)
to: (end time) (e.g.1702856314)
Returns candles for specified market.
{
"t": [
"1702592100",
"1702593000",
"1702593900",
...
],
"o": [
"0.000990000000000000",
"0.000990000000000000",
"0.000960000000000000",
...
],
"c": [
"0.000980000000000000",
"0.000970000000000000",
"0.000990000000000000",
...
],
"h": [
"0.000990000000000000",
"0.001000000000000000",
"0.000990000000000000",
...
],
"l": [
"0.000960000000000000",
"0.000950000000000000",
"0.000960000000000000",
...
],
"s": "ok"
}
# Networks
GET /networks
Returns available networks information for currencies.
[
"id": 2,
"name": "ETH"
},
{
"id": 3,
"name": "ERC-20"
},
...
]
# Private Endpoints
# API Token
An API token allows you to authenticate to interact with our private API Endpoints. API Token can be issued from the User Profile -> API Tokens page.
It needs to be provided with the header request as follows:
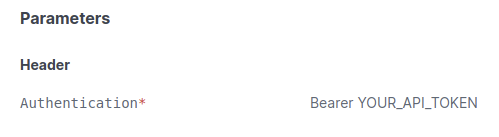
# Get Order
Get Order by Order ID.
GET /orders/getOrder
Parameters:
uuid - Order ID
Successful Response:
{
"data": {
"created_at": "2022-11-08 16:18:08",
"quantity": "1.00000000",
"filled": 0,
"price": "10000.00000000",
"fee": "0.000000000000000000",
"id": "c7a2643c-2a44-4d45-ba81-05fb5d5ff0ab",
"side": "sell",
"type": "limit",
"status": "cancelled"
}
}
Response with an exception:
{
"message": "Order not found"
}
Order Status Types:
active
, filled
, partially_filled
, cancelled
# Place New Order
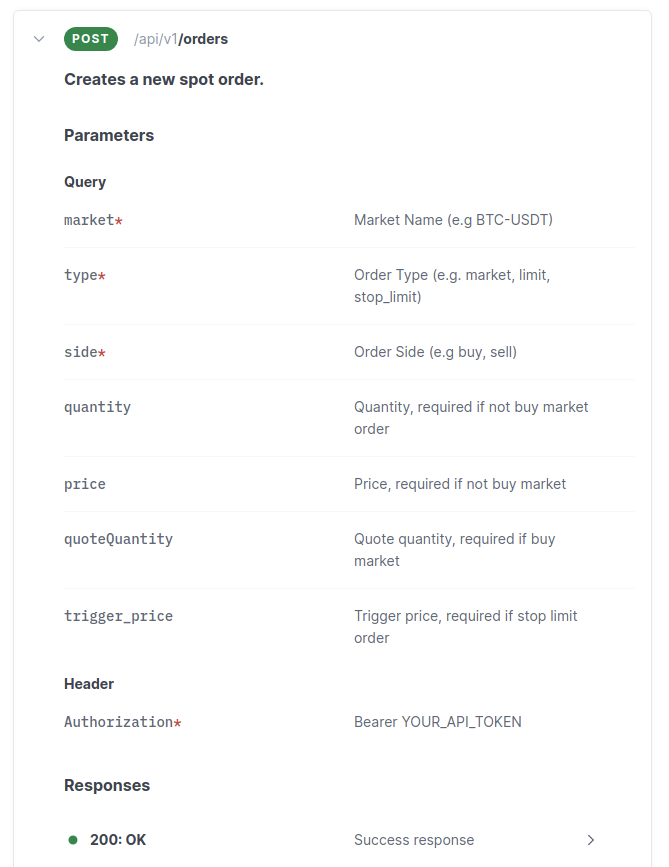
Creates a new spot order.
Successful Response:
{
"message": "cef8aa43-0a7f-44b1-ac2c-6aba8353a456"
}
Response with an exception:
{
"message": "The given data was invalid.",
"errors": {
"quoteQuantity": [
"The quote quantity field is required."
]
}
}
# Cancel Order
Cancels an order.
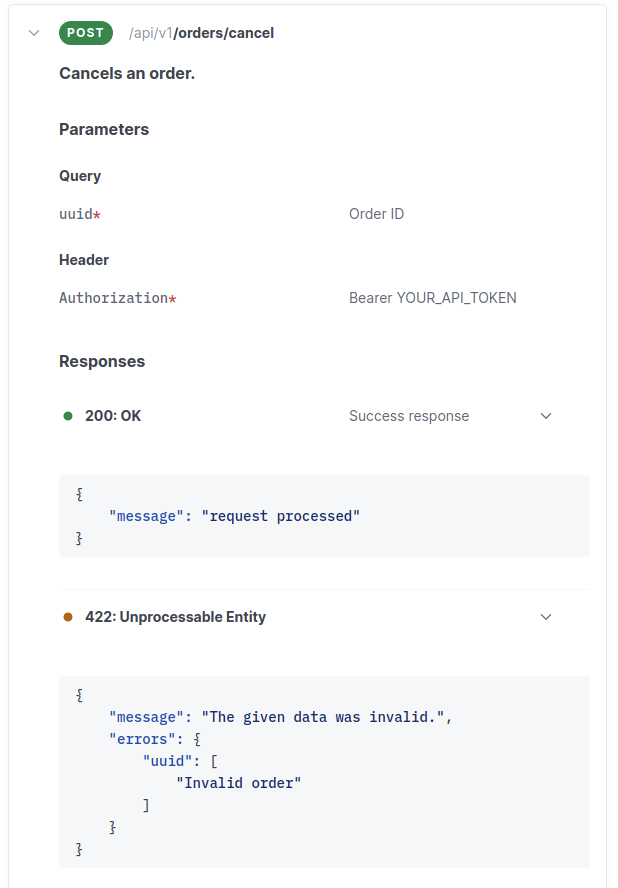
# Open Orders
Returns a list of all active orders.
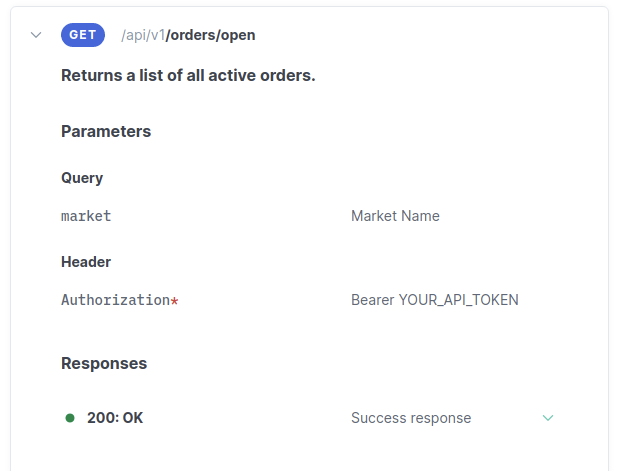
Response:
{
"data": [
{
"created_at": "2022-08-13 12:18:13",
"quantity": "114.57400000",
"price": "523.70000000",
"id": "709241b0-dff2-4c41-b9eb-2217a2d81044",
"side": "sell",
"type": "limit"
},
{
"created_at": "2022-07-13 15:17:05",
"quantity": "142.05200000",
"price": "823.80000000",
"id": "082c6784-23fa-4dd3-a3b0-7dadd3477a45",
"side": "sell",
"type": "limit"
},
...
]
}
# Get User's Filtered Orders
GET /orders/filter
Parameters:
market - Market Name (optional)
status - `filled`, `partially_filled`, `cancelled` (optional)
limit: (e.g. 15) (optional)
startDate: 2021-01-01 09:12:54 (optional)
endDate: startDate: 2021-01-01 09:12:54 (optional)
Successful Response:
{
"data": [
{
"id": "202f0eed-c172-42ad-a8e3-a6c6e8c4004b",
"market": "BTC-USDT",
"quote_currency": "USDT",
"symbol": "limit",
"type": "limit",
"side": "sell",
"price": "1000.00000000",
"status": "cancelled",
~~"transactions": [],~~
"created_at": "2022-01-21 13:19:25"
},
{
"id": "4753b6be-8e41-4961-af38-bbe87bd92364",
"market": "BTC-USDT",
"quote_currency": "USDT",
"symbol": "limit",
"type": "limit",
"side": "buy",
"price": "36680.11000000",
"status": "filled",
"created_at": "2022-01-21 13:24:21""
},
"success": true
}
# Get User Trades
GET /markets/userTrades
Parameters:
market - Market Name (optional)
side - buy or sell (optional)
limit - Limit amount of trades (optional, max: 1000, default: 100)
startDate: 2021-01-01 09:12:54 (optional)
endDate: startDate: 2021-01-01 09:12:54 (optional)
Successful Response:
{
{
"data": [
{
"side": "buy",
"price": "10000.00000000",
"quantity": "0.00000010",
"created_at": "2022-01-15 06:54:05",
"market": "BTC-USDT"
},
{
"side": "sell",
"price": "10000.00000000",
"quantity": "0.00000010",
"created_at": "2023-01-15 06:54:05",
"market": "BTC-USDT"
}
],
"success": true
}
# Get Balances
Returns the user's wallet balances
GET /wallets
Response:
{
"data": [
{
"currency": "Bitcoin",
"symbol": "BTC",
"type": "coin",
"address": "3FZzci21cpjq2GjdwV0uZHuMMnkLkktZb1",
"payment_id": null,
"balance_in_wallet": "3.36366124",
"balance_in_order": "0.01255297",
"balance_in_withdraw": "0.00000000"
},
{
"currency": "Tether",
"symbol": "USDT",
"type": "coin",
"address": "0x19d12c135de20b07b3791f1f61385c193c8e5f33",
"payment_id": null,
"balance_in_wallet": "20000.295140",
"balance_in_order": "1251.918970",
"balance_in_withdraw": "0.000000"
},
{
"currency": "Ethereum",
"symbol": "ETH",
"type": "coin",
"address": "0x19d12c135de20b07b3791f1f61385c193c8e5f33",
"payment_id": null,
"balance_in_wallet": "12.10523164",
"balance_in_order": "1.80823885",
"balance_in_withdraw": "0.00000000"
},
...
],
"success": true
}
# Get Deposits
GET /wallets/deposits/crypto
Returns the user's deposits
Params:
limit: (e.g. 15) (optional)
startDate: 2021-01-01 09:12:54 (optional)
endDate: startDate: 2021-01-01 09:12:54 (optional)
Response:
{
"data": [
{
"deposit_id": "4549860e-c9ba-11ed-afa1-0242ac120002",
"status": "completed",
"txn": "TXN_STRING_HERE",
"amount": "1.01429990",
"fee": 0,
"currency": "Bitcoin",
"symbol": "BTC",
"address": "ADDRESS_HERE",
"payment_id": null,
"confirms": 2,
"created_at": "2022-08-03T11:18:37.311021Z"
}
...
]
}
# Get Deposit Addresses
GET /deposit/address?
Returns the user's addresses with network name
Params:
symbol (e.g. USDT)
Response:
{
[
{
"network": "erc20",
"address": "0x1a89a6b155a40715acd8ea10683254cf402d06cc"
},
{
"network": "trc20",
"address": "TNjJaaK8snRtA1hpozrMm8V6zXFZ7GT2s"
}
]
}
# Get Withdrawals
GET /wallets/withdrawals/crypto
Returns the user's withdrawals.
Params:
limit: (e.g. 15) (optional)
startDate: 2021-01-01 09:12:54 (optional)
endDate: startDate: 2021-01-01 09:12:54 (optional)
Response:
{
"data": [
{
"Withdrawals": "4549860e-c9ba-11ed-afa1-0242ac120002",
"status": "completed",
"txn": "TXN_STRING_HERE",
"amount": "1.01429990",
"fee": 0,
"currency": "Bitcoin",
"symbol": "BTC",
"address": "ADDRESS_HERE",
"rejected_reason": null,
"payment_id": null,
"confirms": 2,
"created_at": "2022-08-03T11:18:37.311021Z"
}
...
]
}
# Withdraw Crypto
POST /wallets/withdraw
Withdraw crypto endpoint. The transaction gets the status Pending right after the creation.
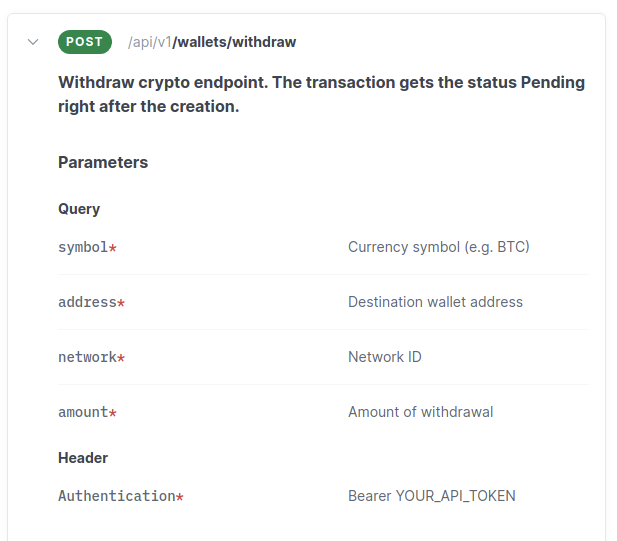
Successful Response:
{
"result": true
}
Response with an exception:
{
"message": "The given data was invalid.",
"errors": {
"amount": [
"Insufficient balance"
]
}
}
← Swap Troubleshooting →